express 코드
import express from 'express' /* const express = require('express');*/ const app = express(); app.get('/posts',function(req,res,next){ res.status(200).send('Hi'); }); app.post('/posts',function(req,res,next){ res.status(200).send('hi'); }); app.put('/posts/:id',function(req,res,next){ res.send(...); }); app.delete('/posts/:id',function(req,res,next){ res.send(...); }); app.listen(8080);
위 코드를 보면, http.createSever() 과 달리 훨씬 간편하게 서버를 만들고 경로를 설정할 수 있다.
express 문법
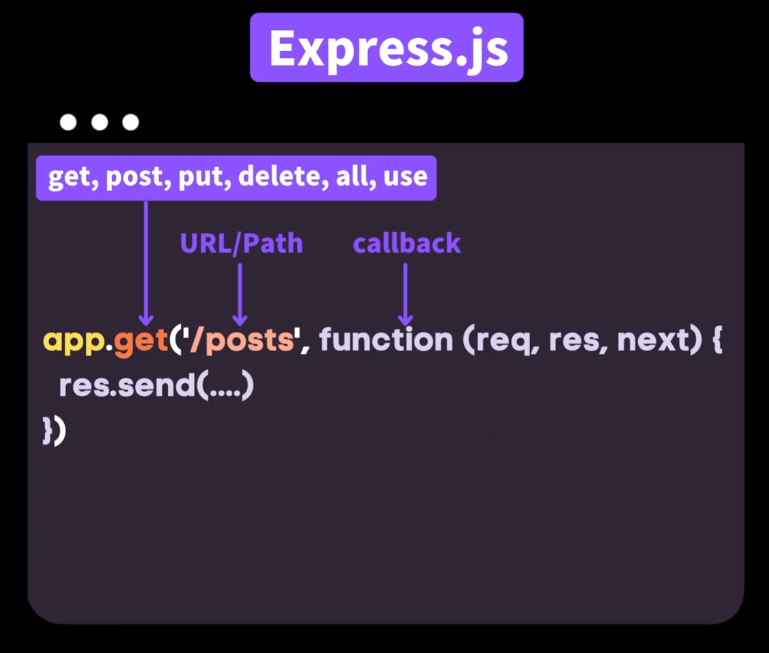
- get 부분은 trequest method와 동일
- 첫번째 인자(URL/Path)
- 어떤 URL에 대해 처리할건지 지정할 수 있음
- 두번째 인자 (콜백)
- /posts 라는 경로에 get이라는 이벤트가 발생하면 요청들어온 오브젝트(req)와 반응할 수 있는 오브젝트(res), 다음으로 넘어갈 수 있는 next가 인자로 들어간다.
- 데이터를 보내고싶다면 res.send()를 호출하면 된다.
이렇게 request를 받고 response를 처리하고, next를 받아서 들어오는 이 함수를 ‘middleware‘ 라고 한다.
express를 한마디로 표현하자면, middleware의 체인이라고 볼 수 있다.
middleware chain
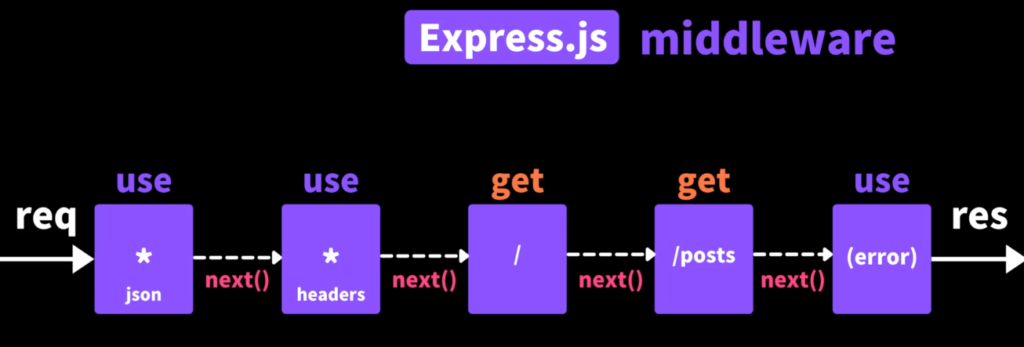
express란, 등록한 미들웨어(콜백함수)의 연속이라 볼 수 있다.
클라이언트가 GET method를 이용하여 / 경로에 request가 접수가 되면, use 미들웨어에서는 json을 파싱한 다음에 next 함수를 호출하고 다음 미들웨어에서는 headers에 대한 부분을 처리하고 다음으로 넘긴다. ‘/’ 경로의 get을 처리하는 미들웨어에서 res.send()를 통해 response를 보내준 뒤, next()를 호출하지 않고 끝낸다.
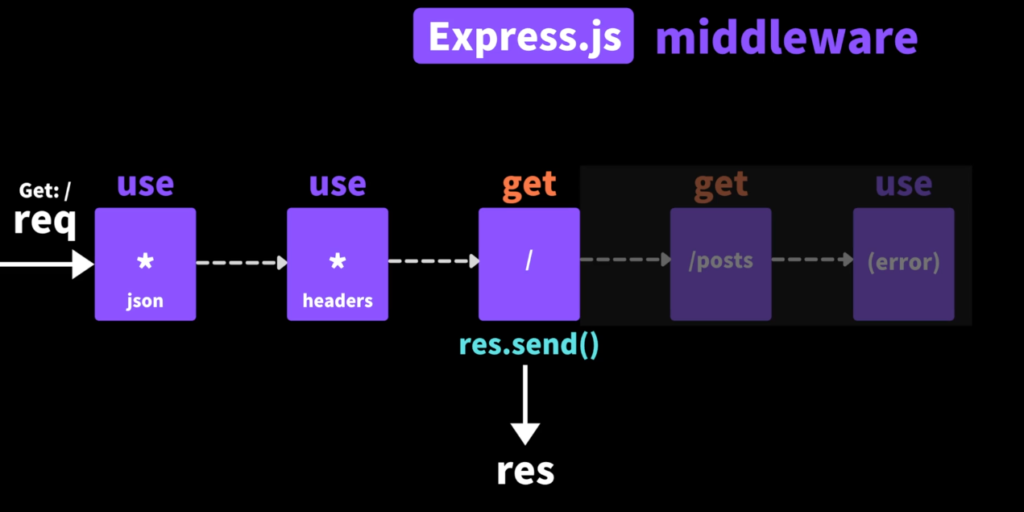
- app.use
- app.use 안에 있는 모든 함수들은 모두 미들웨어이며 요청이 올때마다 이 미들웨어를 거치며 클라이언트에게 응답하게 된다.
- app.get / post / put / delete
- 특정한 경로, 특정한 메서드 요청에 대한 처리를 담당하는 미들웨어
마지막으로 등록된 미들웨어에서 에러를 던지거나 클라이언트에게 응답을 주면 끝! 👏
import express from 'express' const app = express(); app.get('/',(req,res,next) => { // console.log(req.path); // console.log(req.headers); console.log('get!'); console.log(req.query); res.status(200).send('hi!'); }); app.get('/student/:id',(req,res,next) => { // http://localhost:8080/student/dylan?keyword=1 로 요청이 들어올때 console.log(req.params.id); // student 아래에 param값중 id를 받아올 수 있음 (dylan) console.log(req.query.keyword); // ? 뒤의 query string 중 keyword 값을 받아올 수 있음 (1) console.log(req.subdomains); // res.status(200).send('hi!'); // res.json({name:'dylan'}); // json으로 전달 res.setHeader('key','value'); // 헤더에 key - value 쌍으로 생성 res.sendStatus(200); // status를 보냄과 동시에 'OK'를 보내줌 }); app.post('/',(req,res,next)=>{ }); app.listen(8080);
app.use 와 app.all 의차이
use와 all은 어떤 http 요청이든 상관없이 지정한 경로나, 모든 경로에 대해서 콜백함수를 수행해준다. use 와 all은 비슷하지만 살짝 다르다. 아래 포스팅을 참고하자