웹뷰 세가지 중점
- WebView
- AppBar
- pub.dev
- https://pub.dev/packages/webview_flutter/versions/3.0.4
import "dart:io"; import "package:flutter/material.dart"; import "package:webview_flutter/webview_flutter.dart"; class HomeScreen extends StatelessWidget { const HomeScreen({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Dylan test'), // app bar 타이틀 centerTitle: true, // 타이틀 중앙 정렬 backgroundColor: Colors.black, ), body: WebView( initialUrl: 'https://mingsayz.com', javascriptMode: JavascriptMode.unrestricted, // js 사용 가능 (기본적으론 disabled 임) ), ); } }
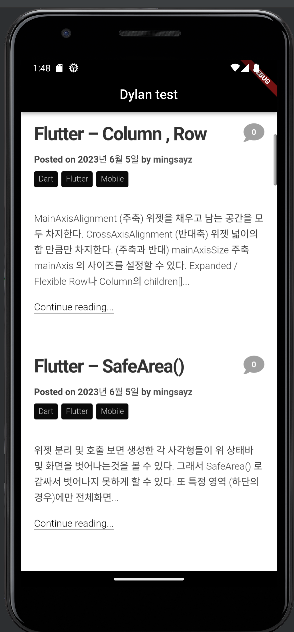
Controller
특정 이벤트를 컨트롤 할 수 있는 컨트롤러를 등록하고, 이벤트 발생시 홈으로 리다이렉트하는 버튼을 만들어보자
import "dart:io"; import "package:flutter/material.dart"; import "package:webview_flutter/webview_flutter.dart"; class HomeScreen extends StatelessWidget { WebViewController? controller; // controller 선언 final homeUrl = 'https://mingsayz.com'; HomeScreen({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Dylan test'), centerTitle: true, backgroundColor: Colors.black, actions: [ IconButton( onPressed: () { if(controller == null){ return; } controller!.loadUrl(homeUrl); // controller 는 null 이 될수없음 (!) }, icon: Icon(Icons.home), ), ], ), body: WebView( onWebViewCreated: (WebViewController controller) { this.controller = controller; // webview가 생성될때 이벤트를 controller에 보내도록 등록 }, initialUrl: homeUrl, javascriptMode: JavascriptMode.unrestricted, // js 사용 가능 ), ); } }
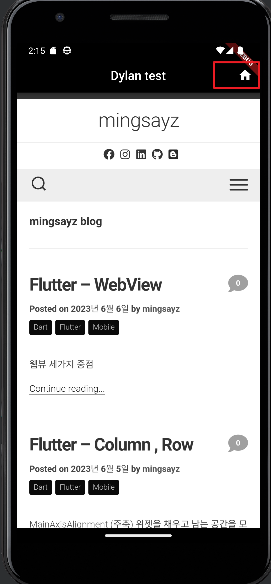
HTTP 프로토콜 사용하고 싶다면?
기본적으로 모바일앱은 HTTP 프로토콜 사용을 막고 있다.
사용하고싶다면 각 플랫폼별로 코드를 추가해줘야한다.
ios (/ios/Runner/Info.plist)
<key>NSAppTransportSecurity</key> <dict> <key>NSAllowsLocalNetworking</key> <true/> <key>NSAllowArbitraryLoadsInWebContent</key> <true/> </dict>
android (/android/app/src/main/AndroidManifest.xml)
<manifest xmlns:android="http://schemas.android.com/apk/res/android"> <uses-permission android:name="android.permission.INTERNET"/> <!--안드로이드 인터넷 퍼미션 넣어줘야함 --> <application android:name="${applicationName}" android:icon="@mipmap/ic_launcher" android:label="webview_example" android:usesCleartextTraffic="true"> <!--http 사용가능하게 하기위해 삽입하는 코드--> <activity android:name=".MainActivity" android:configChanges="orientation|keyboardHidden|keyboard|screenSize|smallestScreenSize|locale|layoutDirection|fontScale|screenLayout|density|uiMode" android:exported="true" android:hardwareAccelerated="true" android:launchMode="singleTop" android:theme="@style/LaunchTheme" android:windowSoftInputMode="adjustResize"> <!-- Specifies an Android theme to apply to this Activity as soon as the Android process has started. This theme is visible to the user while the Flutter UI initializes. After that, this theme continues to determine the Window background behind the Flutter UI. --> <meta-data android:name="io.flutter.embedding.android.NormalTheme" android:resource="@style/NormalTheme" /> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <!-- Don't delete the meta-data below. This is used by the Flutter tool to generate GeneratedPluginRegistrant.java --> <meta-data android:name="flutterEmbedding" android:value="2" /> </application> </manifest>
WebView 4.x 버전 대응
import "package:flutter/material.dart"; import "package:webview_flutter/webview_flutter.dart"; final homeUrl = Uri.parse('https://mingsayz.com'); class HomeScreen extends StatelessWidget { WebViewController controller = WebViewController() ..setJavaScriptMode(JavaScriptMode.unrestricted) ..loadRequest(homeUrl); // controller 선언 HomeScreen({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Dylan test'), centerTitle: true, backgroundColor: Colors.black, actions: [ IconButton( onPressed: () { controller.loadRequest(homeUrl); // controller 는 null 이 될수없음 (!) }, icon: Icon(Icons.home), ), ], ), body: WebViewWidget( controller: controller, ), ); } }