1. Sequelize ORM DB 연결 테스트
// database.js import { config } from "../config.js"; //import mysql from "mysql2"; import SQ from "sequelize"; const { host, user, database, password } = config.db; export const sequelize = new SQ.Sequelize(database, user, password, { host, dialect: "mysql", });
// app.js sequelize.sync().then((client) => { const server = app.listen(config.host.port); initSocket(server); }); // DB가 잘 연결된 다음, 서버 실행 (sequelize는 자동으로 로그를 기록한다) 나중에 꺼주도록 하겠음!
2. 테이블 생성 / 정의
import { db, sequelize } from "../db/database.js"; import SQ from "sequelize"; const DataTypes = SQ.DataTypes; const User = sequelize.define("user", { id: { type: DataTypes.INTEGER, autoIncrement: true, allowNull: false, primaryKey: true, }, username: { type: DataTypes.STRING(45), allowNull: false, }, password: { type: DataTypes.STRING(128), allowNull: false, }, name: { type: DataTypes.STRING(128), allowNull: false, }, email: { type: DataTypes.STRING(128), allowNull: false, }, url: DataTypes.TEXT, });
Sequelize 를 연결하면, 자동으로 로그가 기록된다. 테이블이 없다면 자동으로 생성시킨다는 로그를 볼 수 있다 🙂
로그를 남길 필요가 없다면 Sequelize 정의시, logging:false 를 주면 된다.

시퀄라이즈가 테이블 스키마 생성 / 정의 해줬음을 볼 수 있다. (createdAt 과 updatedAt 은 자동 생성됨)
만약 timestamp 가 필요없다면, define() 시 인자로 timestamps: false 를 넘기면 된다.
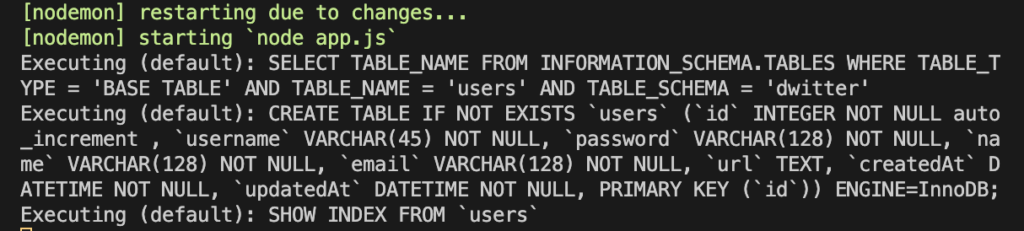
3. user 찾기 / 생성 수정
기존 ‘https://mingsayz.com/?p=3117’ 에서 mysql 만을 이용해서 작성했던 코드와 비교해보자 !
export async function findByUsername(username) { return User.findOne({ where: { username } }); } export async function findById(id) { return User.findByPk(id); } export async function createUser(user) { return User.create(user).then((data) => data.dataValues.id); }
4. 전체 코드 수정
import { sequelize } from "../db/database.js"; import SQ from "sequelize"; import { User } from "./auth.js"; const DataTypes = SQ.DataTypes; const Sequelize = SQ.Sequelize; const Tweet = sequelize.define("tweet", { id: { type: DataTypes.INTEGER, autoIncrement: true, allowNull: false, primaryKey: true, }, text: { type: DataTypes.TEXT, allowNull: false, }, }); Tweet.belongsTo(User); const INCLUDE_USER = { attributes: [ "id", "text", "createdAt", "userId", [Sequelize.col("user.name"), "name"], [Sequelize.col("user.username"), "username"], [Sequelize.col("user.url"), "url"], ], include: { model: User, attributes: [], }, }; const ORDER_DESC = { order: [["createdAt", "DESC"]], }; export async function getAll() { return Tweet.findAll({ ...INCLUDE_USER, ...ORDER_DESC }); } export async function getAllByUsername(username) { return Tweet.findAll({ ...INCLUDE_USER, ...ORDER_DESC, include: { ...INCLUDE_USER.include, where: { username }, }, }); } export async function getById(id) { return Tweet.findOne({ where: { id }, ...INCLUDE_USER, }); } export async function create(text, userId) { return Tweet.create({ text, userId }).then((data) => this.getById(data.dataValues.id) ); } export async function update(id, text) { return Tweet.findByPk(id, INCLUDE_USER).then((tweet) => { tweet.text = text; return tweet.save(); }); } export async function remove(id) { return Tweet.findByPk(id).then((tweet) => { tweet.destroy(); }); }